This project – actually a mini project – might also be interesting for one or the other. It is the now well-known and frequently used carbon dioxide sensor SCD30 (CO2 sensor) from the manufacturer Sensirion. There are a number of projects that can be found on the internet. As part of a quick test setup, I tried to read out the data from the sensor using an Arduino Uno board in order to then display it in a plot using the Matlab software. The data transfer takes place via the serial interface or via the serial protocol of the USB-UART.
To connect the SCD30 to the Arduino, you need the power supply and the I²C data bus – so in total just four wires. This means that the minimum configuration is fulfilled and the data can be read out.
The sensor itself works on the principle of NDIR technology. (NDIR = non-dispersive-infrared). That means the sensor is a small spectrometer. The medium to be examined is fed into a sample chamber. The sample chamber is illuminated by an infrared source and the IR light shines through the medium and a very narrow-band wavelength filter and then hits the IR detector. The wavelength of the filter is designed in such a way that precisely those wavelengths are let through that are absorbed by the molecules of the medium (gas). Depending on the number of molecules or the density of the gas, fewer light beams are recognized by the detector. A second measuring chamber, which is filled with a reference gas, serves as a reference. A controller on the sensor evaluates this information and forwards it in the form of ppm via the I²C (or switchable MOD-Bus) interface. There is also a temperature and humidity sensor on the board, the data of which can also be read out via the bus. The preset I²C address of the SCD30 is 0x61. The exact information on the data protocol can be found in the documentation from Sensirion.
Ideally, as almost always, there is already a ready-made library suitable for students for the various microcontrollers. So you don’t have to worry anymore and can read out the data from the connected sensor directly. The example programs can be found under the examples of the libraries.
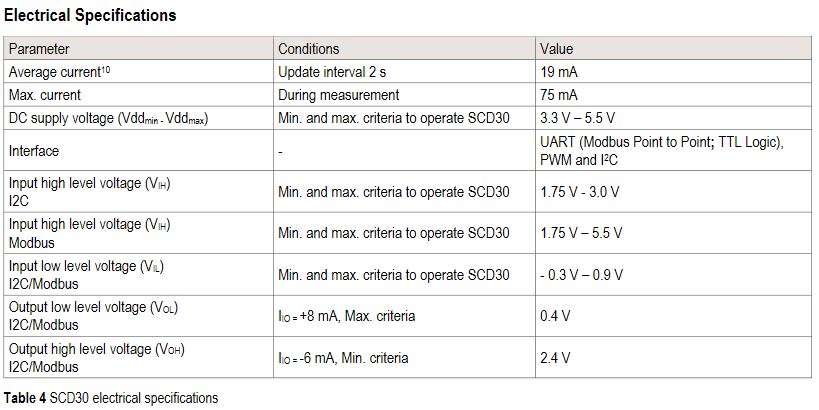
The Arduino with 3.3V or 5V can be used for the supply voltage of the sensor. However, caution is advised when using the I²C bus: Here the input high level is set at 1.75-3.0V and the output high level with a maximum of 2.4V. But on an Arduino the levels are 5V !! So a level shifter has to be built in here – or at least, suitable resistors for a quick test.
The program code listed here essentially comes from the example of the library by Nathan Seidle from SparkFun Electronics:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
/* By: Nathan Seidle SparkFun Electronics Library: http://librarymanager/All#SparkFun_SCD30 */ #include <Wire.h> #include "SparkFun_SCD30_Arduino_Library.h" SCD30 airSensor; void setup() { Wire.begin(); Serial.begin(9600); //Serial.println("SCD30 Example"); airSensor.begin(); //This will cause readings to occur every two seconds } void loop() { if (airSensor.dataAvailable()) { // Serial.print("co2(ppm):"); Serial.print(airSensor.getCO2()); //Serial.print(" temp(C):"); Serial.print(","); Serial.print(airSensor.getTemperature(), 1); // Serial.print(" humidity(%):"); Serial.print(","); Serial.print(airSensor.getHumidity(), 1); Serial.println(); } else //Serial.println("No data"); delay(500); } |
With these lines of code in the Arduino Uno and the correct wiring (SDA -> to Arduino A4 and SCL -> to Arduino A5 via a suitable level converter) you can continue with Matlab. The Arduino should now output the following lines in a serial terminal: (example)
473,28.5,12.9
473,28.5,13.0
470,28.5,13.1
469,28.5,12.9
466,28.5,12.9
465,28.5,12.7
465,28.5,12.5
463,28.6,12.6
461,28.6,12.5
463,28.5,12.4 … und so weiter
This now has to be read into Matlab and recorded over a definable period and at the same time displayed in a plot. The matlab script here makes it possible … (pn if someone needs it)
The result is a plot that shows the course of CO2 in the room (in this case on my office desk).